Overview:
对象和类,面向对象思维,多态与继承
对象和类,面向对象思维,多态与继承
41.Review:
鬼灭之刃主题教学
Instance Variable实例变量
A swordsman has a name 记录鬼灭剑客的各项数据
○ we also want to keep track whether they are alive,
and the number of demons they have killed
○ we also want to keep track whether they are alive,
and the number of demons they have killed
public class Swordsman {
private String name;
private boolean alive;
private int numDemonsKilled;
...
}
Constructor 构造器
接上...的内容
Error example
public Swordsman(String n) {
String name = n;
boolean alive = true;
int numDemonsKilled = 0;
}
Right example
public Swordsman(String name) {
this.name = name;
alive = true;
numDemonsKilled = 0;
}
Test Client, Object Instantiation 测试客户端,对象实例化
接上...的内容
public static void main(String[] args) {
Swordsman tanjiro = new Swordsman("Tanjiro");
}
Reference Type 引用类型
Private 私有变量
接上...的内容
By setting the instance variables to be private, client classes
实例变量设置为私有的
其他方法 cannot directly access or change their values
By setting the instance variables to be private, client classes
实例变量设置为私有的
其他方法 cannot directly access or change their values
Compile error
public class Swordsman {
private String name;
...
}
public class DemonSlayerCorps {
...
public static void main(String[] args) {
Swordsman tanjiro = new Swordsman("Tanjiro");
tanjiro.name = "Inosuke";
}
}
ToString() Method
toString() is a method that returns string representation of this object.
○ it enables an object to be printed 是一种返回此对象的字符串表示形式的方法,字面意思。
○ it enables an object to be printed 是一种返回此对象的字符串表示形式的方法,字面意思。
public class Swordsman {
...
public String toString() {
String alive;
if (this.alive) alive = "alive";
else
alive = "dead";
return "Swordsman " + name + " is " + alive +
" and has killed " + numDemonsKilled + " demons";
}
automatically calls
tanjiro.toString()
Getter and Setter
get+object() 区别是get取得了一个值,set+object() set构建了一个值
public class Swordsman {
private String name;
...
public String getName() {
return name;
}
public void setName(String newName) {
name = newName;
}
...
}
Instance Method
Instance method performs operation on instance variables of the instance.
○ Instance method is called on an object
用法:实例对象.实例方法
○ Instance method is called on an object
用法:实例对象.实例方法
public class Swordsman {
private int numDemonsKilled;
...
public void killsDemon() {
numDemonsKilled++;
}
public static void main(String[] args) {
Swordsman tanjiro = new Swordsman("Tanjiro");
tanjiro.killsDemon();
System.out.println(tanjiro);
}
}
Method Overloading
Method overloading: same name, different signature
○ same method name, different parameter numbers or types
一个方法名字不同的参数编号或类型
○ same method name, different parameter numbers or types
一个方法名字不同的参数编号或类型
public class Swordsman {
private int numDemonsKilled;
...
public void killsDemon() {
numDemonsKilled++;
}
public void killsDemon(int demonsKilled) {
numDemonsKilled += demonsKilled;
}
...
}
Constructors Overloading
same name, different signature
○ we can call other constructors by using this()
一个构造函数也可以被多次重载(overload)为同样名字的函数
○ we can call other constructors by using this()
一个构造函数也可以被多次重载(overload)为同样名字的函数
public class Swordsman {
public Swordsman(String name) {
this.name = name;
alive = true;
numDemonsKilled = 0;
}
public Swordsman(String name, int numDemonsKilled) {
this(name);
this.numDemonsKilled = numDemonsKilled;
}
public Swordsman() {
this("Nameless");
}
}
Static Method / Function
Just like instance methods, it can access and modify instance variables
○ however, a static method is not necessarily called on an object
○ however, a static method is not necessarily called on an object
public static boolean sameNumDemonsKilled(Swordsman s1, Swordsman s2) {
return s1.numDemonsKilled == s2.numDemonsKilled;
}
public static void main(String[] args) {
Swordsman tanjiro = new Swordsman("Tanjiro");
tanjiro.killsDemon();
Swordsman zenitsu = new Swordsman("Zenitsu", 1);
boolean sameKills = sameNumDemonsKilled(tanjiro, zenitsu);
System.out.println(sameKills);
}
Class Variable / Static Variable
public class Swordsman {
private String name;
private boolean alive;
private int numDemonsKilled;
private static int numSwordsman = 0;
public Swordsman(String name) {
this.name = name;
alive = true;
numDemonsKilled = 0;
numSwordsman++;
}
}
Accessing Class Variable
To access a class variable, we can use both static or instance methods
public class Swordsman {
public static int getNumSwordsman() {
return numSwordsman;
}
public int getNumSwordsmanInst() {
return numSwordsman;
}
public static void main(String[] args) {
...
System.out.println(getNumSwordsman());
System.out.println(tanjiro.getNumSwordsmanInst());
}
}
Array of Objects
Just like int or String, we can create an array of Swordsman objects
可以为对象创建数组
public class DemonSlayerCorps {
public static void main(String[] args) {
Swordsman[] swordsmanTroop = new Swordsman[2];
swordsmanTroop[0] = new Swordsman("Tanjiro");
swordsmanTroop[1] = new Swordsman("Zenitsu", 1);
for (int i = 0; i < swordsmanTroop.length; i++) {
System.out.println(swordsmanTroop[i]);
}
}
}
经过这轮复习,你掌握了吗?
Overriding&Overloading&Overflowing的区别
覆盖和重载、和溢出
重载(overloading)是指在同一类中定义多个同名但参数类型或数量不同的方法,以实现不同的功能。在编译时根据传递的参数类型和数量确定调用哪个方法。重载方法必须具有不同的参数列表。重载通常用于提供多种不同的方法签名,以方便程序员使用。
覆盖(overriding)是指在子类中定义与父类中同名、参数类型和数量相同的方法,以覆盖父类中的方法。覆盖方法必须具有相同的方法签名(即方法名称、参数类型和返回类型)。覆盖方法通常用于实现多态性(polymorphism),即同一个方法调用可以在不同的对象上产生不同的行为。
重载(overloading)是指在同一类中定义多个同名但参数类型或数量不同的方法,以实现不同的功能。在编译时根据传递的参数类型和数量确定调用哪个方法。重载方法必须具有不同的参数列表。重载通常用于提供多种不同的方法签名,以方便程序员使用。
public class MathUtils {
public int add(int x, int y) {
return x + y;
}
public double add(double x, double y) {
return x + y;
}
}
覆盖(overriding)是指在子类中定义与父类中同名、参数类型和数量相同的方法,以覆盖父类中的方法。覆盖方法必须具有相同的方法签名(即方法名称、参数类型和返回类型)。覆盖方法通常用于实现多态性(polymorphism),即同一个方法调用可以在不同的对象上产生不同的行为。
public class Animal {
public void speak() {
System.out.println("I am an animal.");
}
}
public class Cat extends Animal {
@Override
public void speak() {
System.out.println("Meow!");
}
}
溢出(overflowing)是指计算或操作时结果超出了数据类型的表示范围而发生数字溢出的现象。当进行数值运算时,如果结果超出了数据类型所能表示的最大值或最小值,就会发生溢出。
int a = 2_000_000_000;
int b = 2_000_000_000;
int sum = a + b;
System.out.println(sum); // 输出为 -294967296
在这个例子中,尽管 a 和 b 都是 int 类型并且看起来是正常的整数,但是由于它们的总和超出了 int 类型的最大值,因此发生了溢出。结果 -294967296 并不是正确的数值。
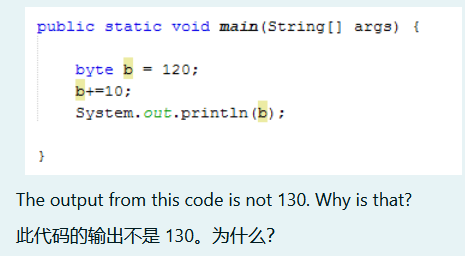
Comments NOTHING