//从这节课开始,课程内容异常抽象,请尽量适应。
不理解可以参考b站视频P64-70,至少能把代码写出来。
不理解可以参考b站视频P64-70,至少能把代码写出来。
40.Object Oriented Programming
2.Primitive data types
(int, double, boolean etc.) store an actual value
什么是主类型(Primitives data types)
什么是主类型(Primitives data types)
3.Primitives have no methods
○ Only the value
什么是原语(Primitives)?
4. Wrapper Classes包装类
Integer, string... are primitives data type. But We can wrap the primitive to access useful methodsAlthough an int is a primitive, an Integer allows us to treat it as an object, and so there are a number of methods, such as Compare() toString() etc. that we can access.
For example,”Integer.toString(3)”contains "wrapper class"(integer), "method"(toString)"parameter"((3)).
”Integer.toString(3)”
In this code,
the Integer class is used to encapsulate the integer value 3 as an objectand then
convert it to a string representation by calling the toString method.This encapsulation
allows integers to be used like objects because they inherit from the Object class, which is the base class for all classes in Java. This encapsulation allows you to perform operations where objects are needed, such as putting integers into a collection class or passing them to methods that require object arguments.5.Object的理解
//开始晕头转向了?加大力度!
● Objects (String, Scanner, Math) are referred to by reference
○ The variable holds a memory address pointing to the object
● The object contains properties (variables) and behaviour (methods)
These objects have state and behaviour
●key points
Can store everything we need in objects
Objects have Attributes - variables and Methods
Anything can be an object!
Better to group connected data together as an object
Objects
have methods● Objects (String, Scanner, Math) are referred to by reference
○ The variable holds a memory address pointing to the object
● The object contains properties (variables) and behaviour (methods)
These objects have state and behaviour
●key points
Can store everything we need in objects
Objects have Attributes - variables and Methods
Anything can be an object!
Better to group connected data together as an object
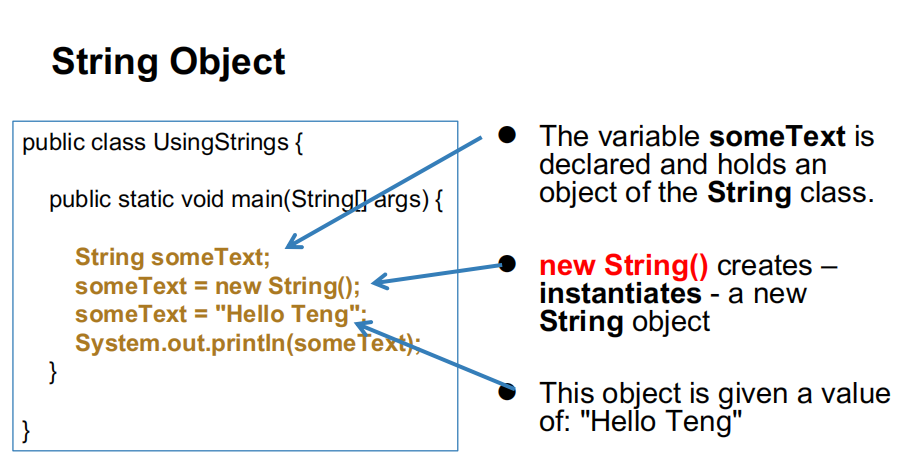
6.String Methods
● myString.length(); 数字
● myString.toLowerCase(); 小写字母
● myString.toUpperCase(); 大写字母
● myString.equals(anotherString); 是否
● myString.equalsIgnoreCase(anotherString); 是否
● myString.contains("java"); 是否
● myString.toLowerCase(); 小写字母
● myString.toUpperCase(); 大写字母
● myString.equals(anotherString); 是否
● myString.equalsIgnoreCase(anotherString); 是否
● myString.contains("java"); 是否
7.Data Objects
Classes in Java ● Currently we have been working in a single class ○ Everything is static, a static class ○ This means that a class is just a class ○ When you run it, there’s one version of it ● However, we can use the same class to create multiple object8.Instance variables
The Person class
Each Person object is an
instance of the Person class
● These variables are called
instance variables
9.包的引用
package
The package refers to a group of java classes.
Constructors
Creating a Person Object
Can be used to assign values when an object is created (“constructed”)
类的抽取 抽出共性的特征和行为,保留所关注的部分。
The package refers to a group of java classes.
Constructors
Creating a Person Object
Can be used to assign values when an object is created (“constructed”)
类的抽取 抽出共性的特征和行为,保留所关注的部分。
10.Create a constructor
● In our Person class, we created a number of class variables
● But we did not give them values
● As they are objects, each one we create can have different variable values
● It is the constructor that allows our initial objects to be different when they are first created!
● This is how we create different people with the same class
● But we did not give them values
● As they are objects, each one we create can have different variable values
● It is the constructor that allows our initial objects to be different when they are first created!
● This is how we create different people with the same class
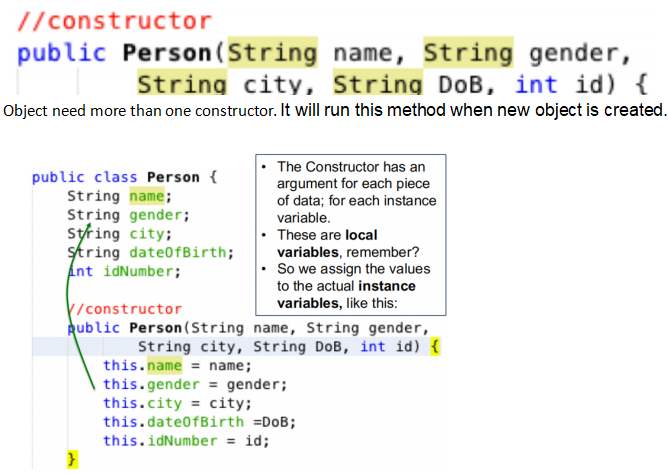
11.This
传递参数和局部变量的定义
Using this.____ refers to this particular object
它可以引用它自己的变量和方法
Can call any of its own methods within the class
或者它自己的任何变量
注意Please use different variable names!
这是一份主方法中的数据
public static void main(String[] args) {
Person p1 = new Person("James Bond", "M",
"London", "1921-11-11", 007);
}
12.Different classes
Main class has no constructor and has many data object.
Other class has a constructor used to construct Person data objects, each with different data. Many different Person data objects will exist.
“static or not” keyword static
● Static means that there is only one instance for the entire class
● So even if we create 100 objects, if we use a static variable for person name, then each person will have the same name
● If it is static, each object will overwrite the previous variable
● Can be useful
Other class has a constructor used to construct Person data objects, each with different data. Many different Person data objects will exist.
“static or not” keyword static
● Static means that there is only one instance for the entire class
● So even if we create 100 objects, if we use a static variable for person name, then each person will have the same name
● If it is static, each object will overwrite the previous variable
● Can be useful
13.Public and Private
If it is PRIVATE, can only be accessed by that class
○ Useful for internal methods
○ something that only needs to be done within the object
○ Useful for internal methods
○ something that only needs to be done within the object
14.Encapsulation
15.Primitive variables and Object variables
原始(基本)数据类型只能表示一个简单的值,不能添加、修改和删除属性。引用数据类型可以包含多个键值对,形成复杂的数据结构,可以动态地添加、修改和删除属性。
原始(基本)数据类型的值是不可变的,引用数据类型的值是可变的。
原始(基本)数据类型的变量赋值是值的复制,而引用数据类型(对象类型)的变量赋值是引用的复制。 Java传递变量的值的方式,传递变量的复本。 当传递引用数据类型时,实际上是将引用的地址传递给了函数。这意味着函数可以通过引用来访问和修改原始对象。引用是指向存储在内存中的对象的地址,所以传递引用时,传递的是指向对象的指针,而不是对象本身的实际值。
此例展示了在 JavaScript 中传递变量的值的方式。
例中定义了一个函数 addTen,它接受一个参数 num。
在此主要关注变量情况:
首先,定义了一个变量 count,并赋值为 20。
接下来,调用 addTen 函数,并将 count 作为参数传递进去。这里需要注意的是,虽然 count 的值是 20,但是在函数中传递的是 count 的值的副本,而不是 count 本身。 在函数内部,num 的值被增加了 10,变成了 30。然后,函数返回了这个新的值,并将其赋给了变量 result。 最后,通过 console.log 打印了 count 和 result 的值。由于在函数中传递的是 count 的值的副本,所以 count 的值没有发生变化,仍然是 20。而 result 的值是函数返回的新值,即 30。
此处虽然抽象,但是也都是编程的通识。
原始(基本)数据类型的值是不可变的,引用数据类型的值是可变的。
原始(基本)数据类型的变量赋值是值的复制,而引用数据类型(对象类型)的变量赋值是引用的复制。 Java传递变量的值的方式,传递变量的复本。 当传递引用数据类型时,实际上是将引用的地址传递给了函数。这意味着函数可以通过引用来访问和修改原始对象。引用是指向存储在内存中的对象的地址,所以传递引用时,传递的是指向对象的指针,而不是对象本身的实际值。
function addTen(num) { // num 是一个局部变量
num += 10;
return num;
}
let count = 20;
let result = addTen(count);
console.log(count); // 20,没有变化
console.log(result); // 30
解析如下:此例展示了在 JavaScript 中传递变量的值的方式。
例中定义了一个函数 addTen,它接受一个参数 num。
在此主要关注变量情况:
首先,定义了一个变量 count,并赋值为 20。
接下来,调用 addTen 函数,并将 count 作为参数传递进去。这里需要注意的是,虽然 count 的值是 20,但是在函数中传递的是 count 的值的副本,而不是 count 本身。 在函数内部,num 的值被增加了 10,变成了 30。然后,函数返回了这个新的值,并将其赋给了变量 result。 最后,通过 console.log 打印了 count 和 result 的值。由于在函数中传递的是 count 的值的副本,所以 count 的值没有发生变化,仍然是 20。而 result 的值是函数返回的新值,即 30。
此处虽然抽象,但是也都是编程的通识。
16.== and .equals()
数字与字符串的不同的等于
17. .toString() method
Java中tostring方法的作用是会返回一个【以文本方式表示】此对象的字符串
“ 什 么 都 有 ”
Now we have a Person data class. It has:
○ Private data fields – instance variables
○ Constructor
○ Public getter methods
○ It is encapsulated
○ No setter methods
○ It is immutable
○ toString() method to print the object's values
Combining with Arrays
● Create an array of 3 people:
Person[] people = new Person[3];
● Instantiate our array of people
● Can use our objects we created earlier, or create new ones
● Now we can navigate through our array similar to before
● For example, if we want to show everyone’s name
“ 什 么 都 有 ”
Now we have a Person data class. It has:
○ Private data fields – instance variables
○ Constructor
○ Public getter methods
○ It is encapsulated
○ No setter methods
○ It is immutable
○ toString() method to print the object's values
Combining with Arrays
● Create an array of 3 people:
Person[] people = new Person[3];
● Instantiate our array of people
● Can use our objects we created earlier, or create new ones
people[0] = p1;
people[1] = p2;
people[2] = new Person("Diana Rigg", "F", "London", "1943-8-
21",2);
For loops ● Now we can navigate through our array similar to before
● For example, if we want to show everyone’s name
for (int i = 0; i < people.length; i++) {
System.out.println("Name is " + people[i].getName());
}
● Can use toString() method to display all
for (int i = 0; i < people.length; i++) {
System.out.println("Name is " + people[i].toString());
}
Comments NOTHING