28.Boolean Operators
The data type that is frequently used called boolean
○ from mathematician George Boole
○ Boolean algebra
Boolean expression 布尔表达式 it just have two values -- true or false.
○ from mathematician George Boole
○ Boolean algebra
Boolean expression 布尔表达式 it just have two values -- true or false.
public class BooleanValues {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println(a);
System.out.println(b);
}
}
Output:
true
false
29.if statement
if(布尔表达式) {
//如果布尔表达式为true将执行的语句
}if(布尔表达式 1){
//如果布尔表达式 1的值为true执行代码
}else if(布尔表达式 2){
//如果布尔表达式 2的值为true执行代码
}else if(布尔表达式 3){
//如果布尔表达式 3的值为true执行代码
}else { //如果以上布尔表达式都不为true执行代码
}
30.While statement
while( 布尔表达式 ) {
//循环内容
}
//先进行布尔表达式的判断,为true,执行循环内容;为false,停止执行循环。
do {
//代码语句
}while( 布尔表达式 );
/*先执行一次循环,再进行布尔表达式的判断,为true,执行循环内容;为false,停止执行循环。*
31.For statement
for(初始化; 布尔表达式; 更新) {
//代码语句
}
For nest //嵌套
public static void main(String[] args) {
System.out.println("乘法口诀表:");
// 外层循环
for (int i = 1; i <= 9; i++) {
// 内层循环
for (int j = 1; j <= i; j++) {
System.out.print(j + "*" + i + "=" + j * i + "\t");
}
System.out.println();
}
}
/*"for-each"循环(也称为增强型for循环或foreach循环)
for(声明语句 : 表达式) { //代码句子 }*/
public class Test {
public static void main(String[] args){
int [] numbers = {10, 20, 30, 40, 50};
for(int x : numbers ){
System.out.print( x );
System.out.print(",");
}
System.out.print("\n");
String [] names ={"James", "Larry", "Tom", "Lacy"};
for( String name : names ) {
System.out.print( name );
System.out.print(",");
}
}
}
32.break
break in loop statement or switch statement ,Used to jump out of the whole sentence block.
break : direcforce exit the cycle
break : direcforce exit the cycle
break;
33.loop
Countdown with While
int countdown = 10; // initialize counter
while(countdown > 0) { // looping condition
System.out.println(countdown);
countdown = countdown - 1; // update
}
System.out.println("Go!");
For Loop Summary
1. The loop is first initialised with a value, which can be any number2. A condition is then checked. If this is met, then the content is processed
3. The next stage is to increment the loop
4. Next, the condition is checked again. If it is still met, then the content is processed again and then incremented
5. This process repeats until the condition is no longer met
34.Store a group
*这里介绍了一些常用的技巧。
Arrays Visualised
Array initialization [计]数组初值,数组预置值
Array Types 数组类型
When created, arrays are automatically initialized
with the default value of their type. E.g.
Arrays Visualised
int[] myArray = new int[5];
myArray[0] = 100; myArray[1] = 200; myArray[2] = 300;
myArray[3] = 400; myArray[4] = 500;
• Arrays have a variable or a property called length
• Here myArray.length will return 5
• However, if you try
myArray[5] = 600;
you will get an error:
ArrayIndexOutOfBoundsExceptionArray initialization [计]数组初值,数组预置值
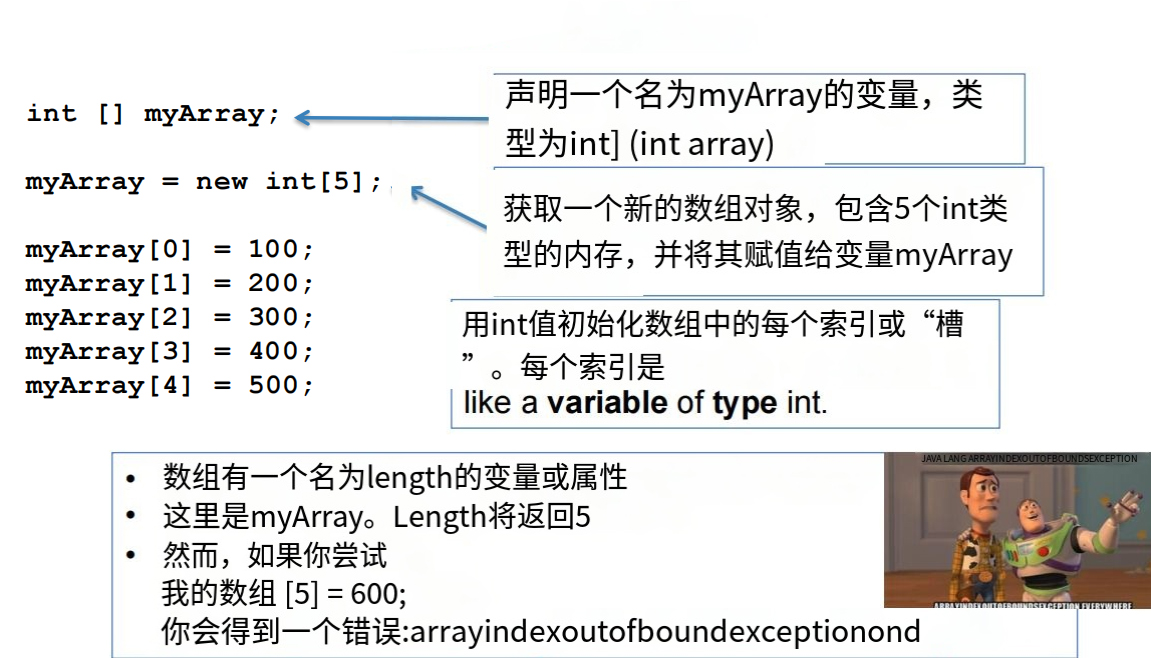
When created, arrays are automatically initialized
with the default value of their type. E.g.
String[] s = new String[100];
// default values: null
boolean[] b = new boolean[4];
// default values: false
int[] i = new int[10];
// default values: 0
Changing an Array Value 更改数组值
String [] myArray = {"a", "b", "c", "d", "e"};
myArray[1] = "hello"; //"a", "hello", "c", "d", "e"
myArray[4] = "bye";//"a", "hello", "c", "d", "bye"
Using an Array Value //使用数组值
String [] myArray = {"a", "b", "c", "d", "e"};
System.out.println("Value is " + myArray[1]);
Value is b
Array Lengths 阵列长度
String [] myArray = {"a", "b", "c", "d", "e"};
int len = myArray.length;
boolean[] myArray = {true, false, true};
int len = myArray.length;
For Loops and Arrays
for (int i = 0; i < myArray.length; i++) {
System.out.println(myArray[i]);
}
Output:a
b
c
d
e
Looping Dice
public static void main(String[] args) {
for(int i = 0; i < 4; i++) {
double rand = ((Math.random() * 6) + 1);
int dice = (int) rand;
System.out.println("Result is " + dice);
}
}
Useful Information Storage
public static void main(String[] args) {
int[] diceList = new int[4];
for(int i = 0; i < 4; i++){
double rand = ((Math.random() * 6) + 1);
int dice = (int) rand;
diceList[i] = dice;
System.out.println("Result is " + dice);
}
}
Find the Total
int total = 0;
for (int i = 0; i < diceList.length; i++) {
total = total + diceList[i];
}
System.out.println("The total is " + total);
35.Methods
Main method
○ What we want out of the method
○ void for nothing
○ int for an integer
○ boolean for a boolean
Used in 2 places
– Declare type in header
– Return a value
Can return nothing
○ Use void
○ Do not return anything
Declare type in header
• Useful for displaying
– otherwise, return the value to
be printed by other methods!
int for an integer
boolean for a boolean
public static void main(String[] args) {
}
Declaring a method
public static int addTwoNumbers() {
/* The first line is the method header */
int result = 3 + 5;
return result;
}
/*Our methods are public static also called static method or function we will explain
this in future weeks for now, don’t worry about this!
公共静态方法
Method must have a name
o (almost) any name you like
o Should be meaningful
o Should tell us what the method does
Declaring
● A method is a code block
● Need to use curly brackets to mark beginning and end */
Declare type in header
Method must return a type○ What we want out of the method
○ void for nothing
○ int for an integer
○ boolean for a boolean
Used in 2 places
– Declare type in header
– Return a value
Return
void for nothingCan return nothing
○ Use void
○ Do not return anything
Declare type in header
• Useful for displaying
– otherwise, return the value to
be printed by other methods!
int for an integer
boolean for a boolean
Calling a method in main
public static void main(String[] args) {
int total = addTwoNumbers();
System.out.println("Total is " + total);
}
public static int addTwoNumbers(){
int result = 3 + 5;
return result;
}
Method Parameter
public static int addTwoNumbers(int num1, int num2) {
int result = num1 + num2;
return result;
}
Calling method with Parameters
public static void main(String[] args) {
int total = addTwoNumbers(3, 5);
displayTotal(total);
}
public static int addTwoNumbers(int num1, int num2) {
/*参数及其类型*/
int result = num1 + num2;
return result;
}
Dice
public static int[] generateDice(int[] input) {
// Loop for length of array
for (int i = 0; i < input.length; i++) {
// Dice generation code
double rand =
((Math.random() * 6) + 1);
int dice = (int) rand;
input[i] = dice;
System.out.println("Result is " +
dice);
}
// return the array
return input;
}
Finding Max Value
public static int findMax(int[] input) {
// Set default max value as being the first value
int max = input[0];
// loop round array, starting from the second value
for (int i = 1; i < input.length; i++) {
// check each value, if greater than current max
if (input[i] > max) {
// set the max to be the new value
max = input[i];
}
}
// return the chosen max value
return max;
}
>>返回总目录<<
Comments NOTHING